Decorators are special functions that can be applied to class declarations, class methods, class attributes and class method parameters to add metadata or modify them in a certain way.
Its easy to notice them as they are usually prefixed with ‘@’ e.g @decoratorMethod
().
They are not only found in Typescript but also in Java and C#. In Javascript they are in stage 2 proposal which means its still a work in progress and hasn’t been released yet. In Typescript it is an experimental feature.
There are a number of frameworks that make use of decorators and these include
Feel free to visit the above amazing frameworks and check out how they are used.
For this article i will mainly focus on decorators in typescript. My assumption is you already know what Typescript is and how to set up a typescript project.
To be able to work with decorators you must make sure it is enabled. Most common way to do this is to edit you tsconfig.ts
file and make sure experimentalDecorators
value has been set to true.

Decorators are one of those topics that are not easy to understand in your first attempt but once you do one or two examples they tend to slowly stick.
Types of decorators
The following are the main types of decorators
- Class Decorators
- Method Decorators
- Accessor Decorators
- Property Decorators
- Parameter Decorators
- Class Decorators
It is placed just before the class definition and it is called during run time. It is used to modify the class it is attached to

In the above our decorator is @MyClassDecorator. Notice that it is declared just before MyClass
definition. This means that we can modify MyClass
using our MyClassDecorator
decorator method.
It is currently empty. Let us add a property called title to it.
First thing is to define the method MyClassDecorator
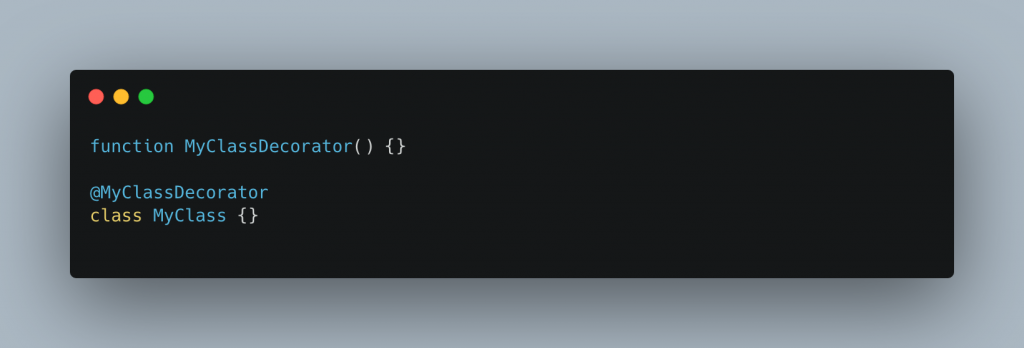
Class decorators receive one argument i.e constructor. We then use that constructor argument to modify our classs

Now we need to make it generic and add generic constraints as Typescript will complain about unknown type being returned
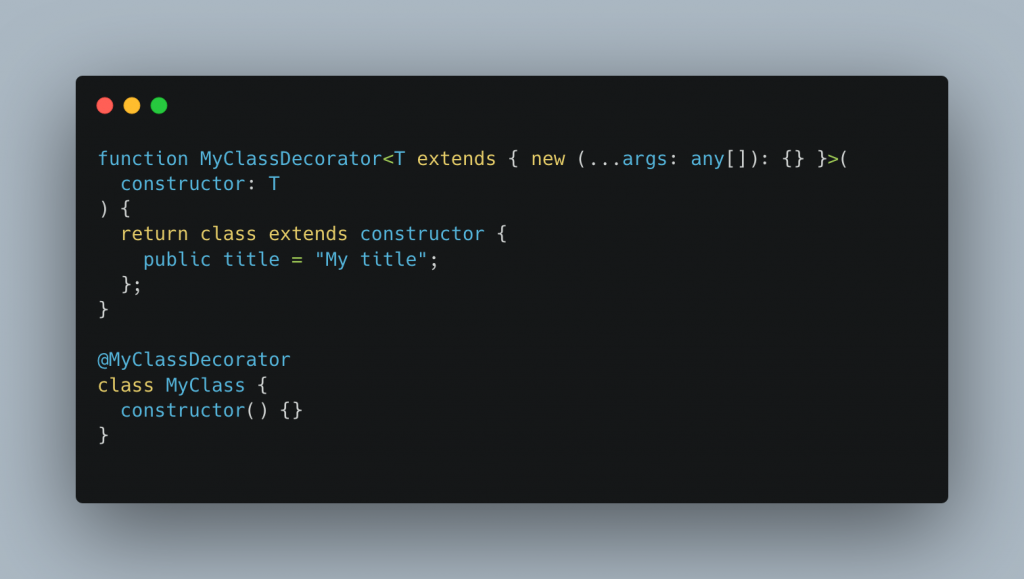
`T extends` simply means we are applying a generic guard and narrowing the generic type to whatever comes after the extends e.g T extends String, T extends object. In our case
`T extends { new (…args: any[]): {}` we are narrowing it down to a generic instance.
Now when we create a new object and call the title property we should be able to see ‘My title’

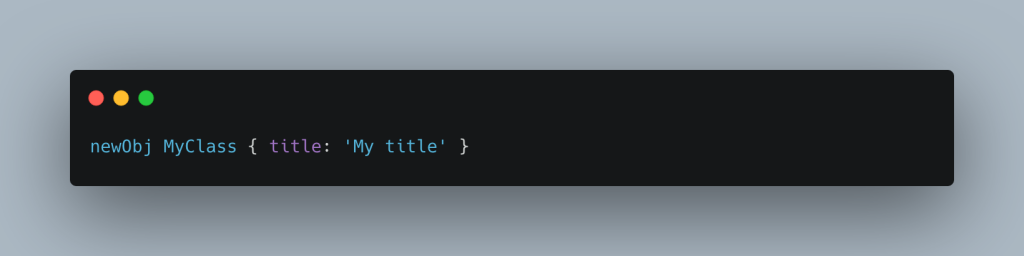
Nice. Lets try add a method that returns the title. Lets call the method getTitle
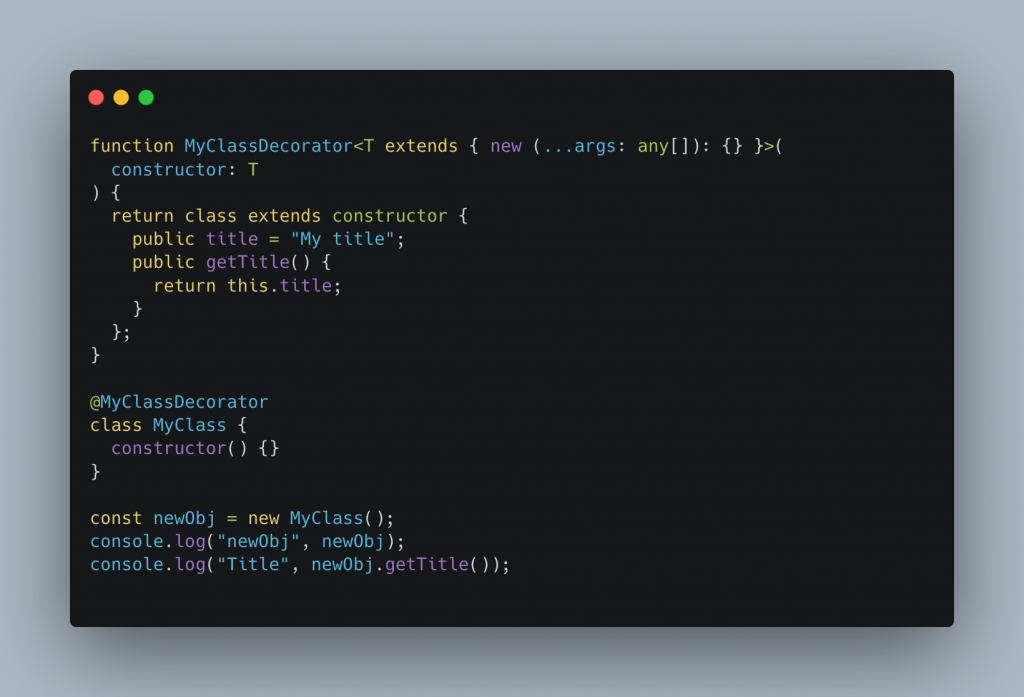
And the output
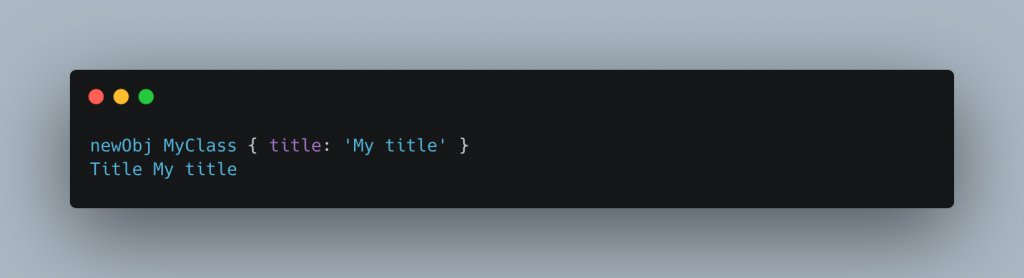
Nice!
I know that’s a lot to take in so for now we’ll stop there and cover method decorators in the next article.